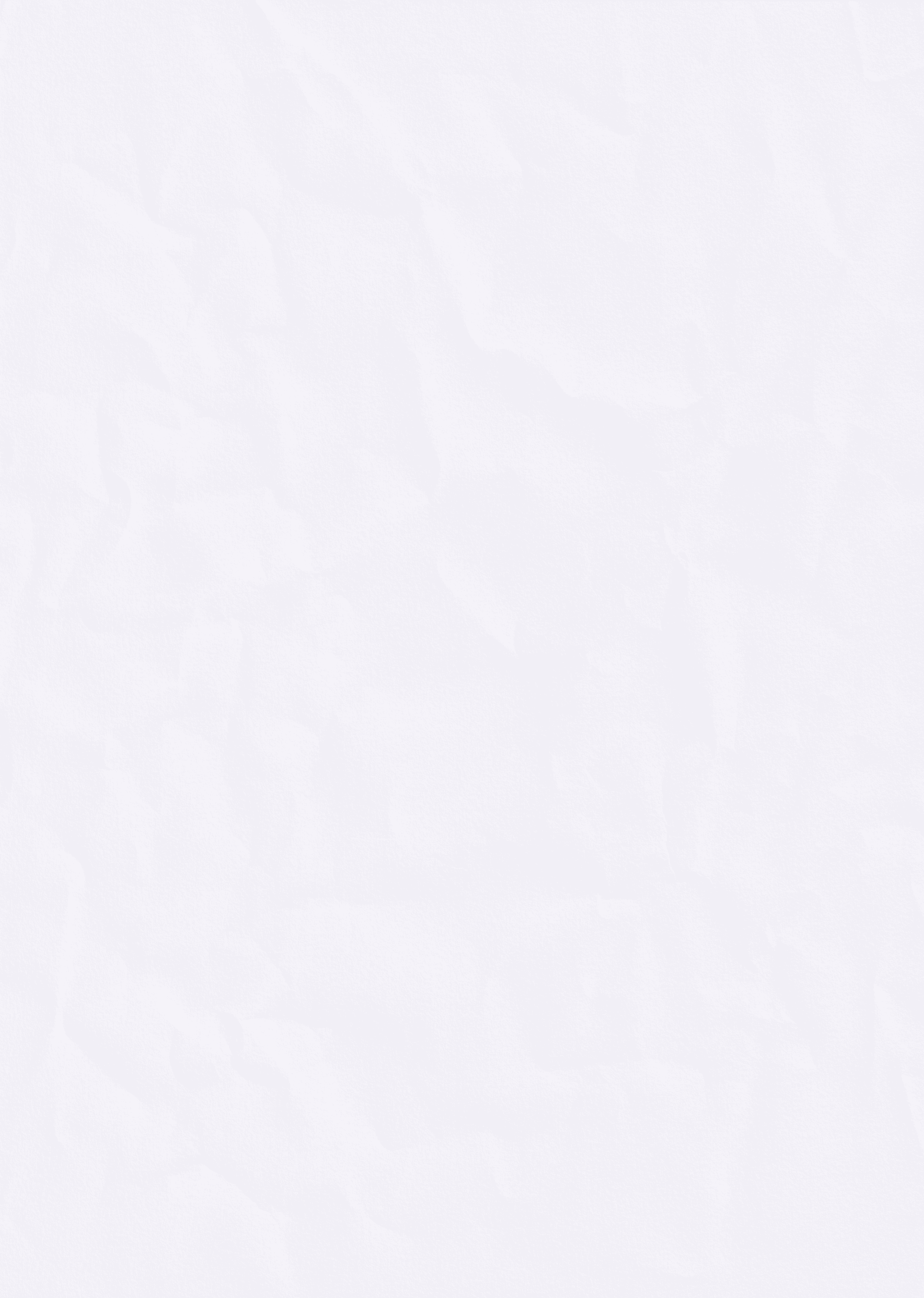
Thershold Based Segmenting // ICM W8
Nov 4, 2024
Assignment & Intent
This project explores the creation of alternative realities through pixel manipulation, using Gianpaolo Tomseri's Mahabharata series as source material. Through threshold-based pixel sorting, I transform these narrative-rich images while diving into the colours used in these culturally significant pieces.
The technical approach uses algorithm-driven pixel sorting with five distinct modes - brightness, gold, pattern, edge, and contrast. This system allows for interactive control over sorting direction and animation speed, while carefully preserving key image elements through threshold-based processing.
In addressing photographic techniques, this method engages with light through brightness and contrast sorting, creates a sense of motion through directional sorting effects, and maintains compositional integrity via threshold-based element preservation. The visual elements are transformed through targeted manipulations: pattern detection highlights architectural details, edge enhancement emphasizes structural elements, gold detection isolates ornamental features, and contrast manipulation establishes new spatial depths.
The content and meaning of the original works remain recognizable while opening new interpretative possibilities. This approach bridges traditional imagery with digital transformation, creating a dialogue between classical storytelling and contemporary manipulation techniques. The resulting transformations can be described in three key words: "Ethereal," "Fluid," and "Transcendent," reflecting the new perspectives offered on these classical images.
Conceptual Framework
Visual Transformation
The heart of this project lies in threshold-based pixel sorting, a technique discovered while exploring the work of digital artists like Kim Asendorf. In 2010, Asendorf pioneered the "pixel sorting" effect through his Processing-based ASDFSort algorithm, which typically created dramatic streaking effects by sorting entire rows or columns of pixels.

Kim Asendorf is a digital artist known for his work with real-time generative systems and his widely recognized Pixel Sorting script.

However, for this project, I took a different approach using threshold-based sorting, which offers more nuanced control by only sorting pixels that meet specific criteria. The key distinction lies in how the sorting is applied. Traditional pixel sorting might dramatically reorganize an entire image, often resulting in the characteristic "glitch art" aesthetic.
In contrast, threshold-based sorting allows us to: - Selectively sort pixels based on specific properties - Preserve important visual elements by setting appropriate thresholds - Target particular features (like gold ornaments or architectural details) - Maintain the overall narrative structure while creating transformation.
This selective approach became particularly crucial when working with Thomasetti's Mahabharata series, where preserving narrative elements while achieving transformation was essential.
Artistic Elements & Image Analysis
In 2003, Giampaolo Thomasetti began a 12-year project creating 25 paintings capturing key moments from the Mahabharata. For this project, I focused particularly on analyzing Images 1 and 5, which showcase distinct characteristics that influenced our sorting parameters:

Giampaolo Thomasetti paintings capturing key moments from the "Mahabharata" epic.

Image 1 ("The Court Scene"):

Strong architectural framing with ornate pillars and archways
Dynamic diagonal composition with figures in motion
Dramatic contrast between light and shadow
Intricate details in clothing and architectural elements
These elements required sorting approaches that could preserve structural integrity through edge detection, enhance the diagonal flow with directional sorting, and maintain dramatic lighting by applying contrast-based sorting.
Image 5 ("The Game"):

Intimate indoor setting with subtle lighting
Horizontal composition with seated figures
Rich fabric textures and patterns
More subdued color palette with focused areas of gold ornamentation
These characteristics required pattern-based sorting to enhance textile details, gold detection to highlight ornamental elements, and careful threshold control to maintain subtle lighting gradations.
The analysis of these images directly influenced the development of our five sorting modes:
Brightness sorting for overall tonal control
Gold detection for ornamental emphasis
Pattern analysis for textile and architectural detail
Edge detection for structural preservation
Contrast sorting for spatial depth
Each mode was designed to respect and enhance different aspects of the original artwork while creating new visual interpretations. This careful balance between transformation and preservation became the guiding principle for the technical implementation that followed.
Technical: Simple to Complex
Foundation: Understanding Threshold-Based Sorting
Before diving into the complex sorting modes, I started to understand the fundamental concept of threshold-based pixel sorting. Unlike traditional pixel sorting that processes entire rows or columns, threshold-based sorting only reorders pixels that meet specific criteria.
Basic Concept:
Each pixel in an image has RGB values (0-255 for each channel)
We calculate a "sorting weight" for each pixel (initially just brightness)
We only sort pixels whose weight exceeds our threshold
This preserves parts of the image while transforming others
This basic implementation demonstrates several key concepts:
Threshold Application
Sorting Weight Calculation
Initially just using average RGB:
(R + G + B) / 3
This became the foundation for more complex weight calculations later
Preservation Through Thresholds
Pixels below threshold remain unchanged
Only "bright" pixels get sorted
This maintains image structure while allowing controlled transformation
This basic version will evolve as I add:
Different sorting modes (brightness, gold, pattern, edge, contrast)
Various sorting directions (vertical, horizontal, diagonal)
Smooth transitions and animation
Interactive controls
Evolution of Sorting Parameters:
Building on our basic threshold-based sorting implementation based on birghtness, I'll show how each sorting mode utilizes the same structural framework but with different approaches to calculating pixel weights.
Contrast Sorting
Edge Detection
Pattern Based
Gold Detection
Advanced Features
What started as a simple threshold-based sorting implementation evolved into a dynamic art tool through several key features:
Directional Flexibility
The ability to sort in different directions expands artistic possibilities. Vertical sorting emphasizes height and architectural elements, while horizontal sorting enhances landscapes and seated figures. Diagonal sorting introduces dynamic flow across the composition, guiding the viewer's eye and adding movement. These techniques provide artists with versatile tools for visual storytelling.
Smooth Transitions
Rather than abrupt pixel movements, we implemented smooth transitions:
This approach creates gradual pixel transitions, enhancing the overall visual flow and providing a more pleasing aesthetic. It also improves the visualization of the sorting process, allowing viewers to better comprehend the changes occurring within the animation. Additionally, it offers control over the animation speed through the 'amp' parameter, enabling fine-tuning of the pacing for a more tailored experience.
Interactive Controls and Layered Effects
This system allows for experimental combination of effects:
This control system allows for real-time switching between sorting modes and the combination of different sorting effects, providing immediate visual feedback. It also includes pause/resume functionality for precise control over the animation.
Performance Optimization
Several strategies ensure smooth performance:
Key optimizations include batch processing of pixels, which improves efficiency by handling multiple pixels at once rather than individually. This is complemented by efficient pixel array handling, allowing for quicker access and manipulation of pixel data. Progress tracking for large images ensures that the sorting algorithm can manage extensive datasets without lag, while smooth animation timing enhances the overall fluidity of the visual output.
The combination of these features transforms the basic sorting algorithm into a versatile artistic tool. Multiple sorting modes can be applied sequentially, allowing for complex visual narratives. Different sorting directions create varied visual effects, adding depth and interest to the animation. Smooth transitions make the process visually engaging, and interactive controls enable users to experiment with different approaches, fostering creativity and exploration within the sorting experience.
Final Outcome
The final outcome is an interactive image processor that enables real-time manipulation of images using various threshold-based pixel sorting techniques. Users can explore visual transformations through a range of sorting modes and interactive controls.
Core Functionality includes handling images of 892x642 pixels and switching between six images from the Mahabharata series while preserving the original state for easy resets. Sorting modes feature brightness, gold detection, pattern enhancement, edge detection, and contrast sorting based on pixel luminosity and color characteristics. Directional controls allow for vertical (default), horizontal, and diagonal sorting with real-time direction switching.
Interactive controls enable users to start or stop processing via mouse clicks and use keyboard shortcuts for toggling sorting modes and images. Visual feedback includes smooth transitions, a progress bar, and console logging of current settings. This setup allows users to experiment with sorting modes, apply multiple passes with varied parameters, and create diverse visual effects. By offering start/stop control, the tool fosters an environment for exploring how different sorting parameters influence image composition while maintaining narrative elements.
<link to final file>
Below is the final Pseudo Code :
Conclusion
This project successfully transformed traditional pixel sorting into an interactive tool for artistic exploration of the Mahabharata series, achieving five distinct sorting modes and implementing threshold-based processing to preserve narrative elements while enabling smooth, controllable transformations. Key technical challenges included efficient pixel processing for large images, creating smooth transitions, developing specific detection algorithms for gold and patterns, and maintaining image integrity during multiple sorting passes. Artistically, the project preserved narrative elements while generating new visual interpretations, highlighted architectural and ornamental details, facilitated fluid transitions between original and transformed states, and encouraged experimental approaches through interactive controls.
Future Possibilities: An Image Encryption Tool
A potentially interesting application for this sorting method could be to use pixel sorting as an encryption mechanism. The concept could work like this:
Imagine an image transformed through a specific sequence of sorting operations:
This sequence creates a unique "key" that combines the sorting modes used, the direction of each sort, the completion percentage of each operation, and the order of operations. This multifaceted key encodes the transformation process, making it integral to both the sorting and decryption methods.
To decrypt the image, you have to apply the exact inverse sequence of the original operations. This involves matching the completion percentages and reversing the sorting directions used during the initial transformation, ensuring that the original image can be accurately restored.
This method introduces a visually interesting form of steganography, where the encrypted image takes on the appearance of an artistic transformation. The decryption key, represented by a sequence of artistic operations, adds an interesting layer to the process, making it both functional and aesthetically engaging.
References
Claude by Anthropic
Ellen for being such a great teacher
https://p5js.org/reference
Ciphrd. "Pixel Sorting on Shader Using Well-Crafted Sorting Filters (GLSL)." Ciphrd, 8 Apr. 2020
Asendorf, Kim. "ASDFSort." GitHub, 2010, https://github.com/kimasendorf/ASDFPixelSort
Reas, Casey, and Ben Fry. "Processing: A Programming Handbook for Visual Designers and Artists." MIT Press, 2014, https://processing.org/handbook/
Menkman, Rosa. "Glitch Art: Deviation From The Norm." Institute of Network Cultures, 2011, https://networkcultures.org/_uploads/NN%234_RosaMenkman.pdf
Nees, Georg. "Schotter." 1968, Computer-generated art, https://collections.vam.ac.uk/item/O221321/schotter-print-nees-georg/
Labbe, Jason. "Processing Pixel Sorting." 2015, https://github.com/JasonLabbe/pixel-sorting
Shiffman, Daniel. "Image Processing with p5.js." The Coding Train, https://thecodingtrain.com/tracks/image-processing-with-p5js
Nake, Frieder. "Algorithmic Art and Aesthetics." Leonardo, Vol. 48, No. 3, 2015
Cascone, Kim. "The Aesthetics of Failure: Post-Digital Tendencies in Contemporary Computer Music." Computer Music Journal, 2000
OpenProcessing - Pixel Manipulation Examples, https://openprocessing.org/browse/?q=pixel%20sorting