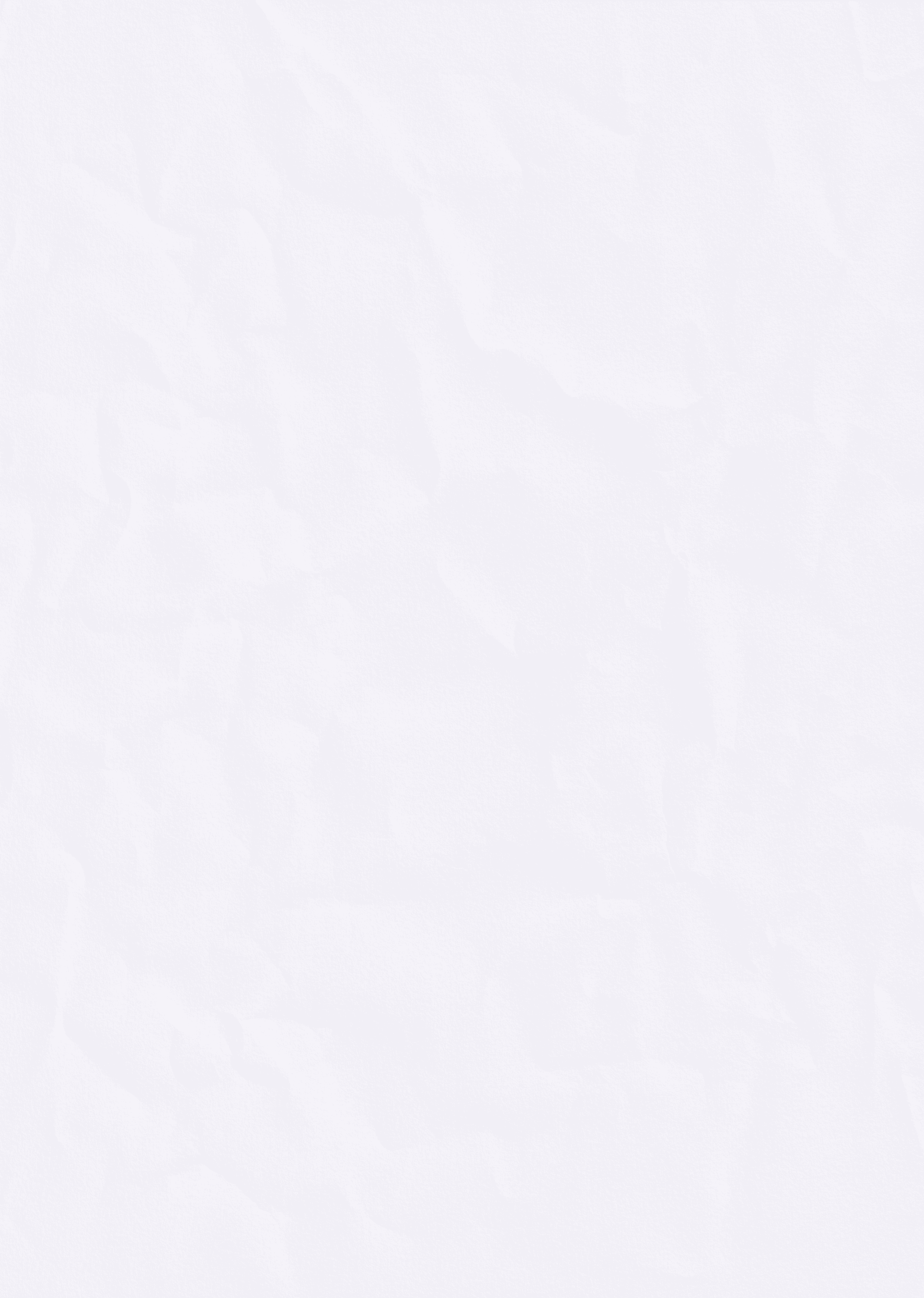
Progress and Regress // ICM W2
Sep 16, 2024
Exploring Opposites
In this blog I'll go through how I explored the ideas of representing a pair of opposite concepts using P5.js. Inspired by Casey Neistat's video, I chose to explore the ideas of progress and regress through interactive motion. Although I found the messaging a little reductive, I really appreciated the way he illustrated the concept in motion.
Concept Overview
My sketch revolves around a circle that moves across the canvas, representing three states:
Staying put
Progressing forward
Regressing backward
The circle's movement is controlled by user interaction, creating a simple yet engaging visualization of progress and regress.
Initial Sketches
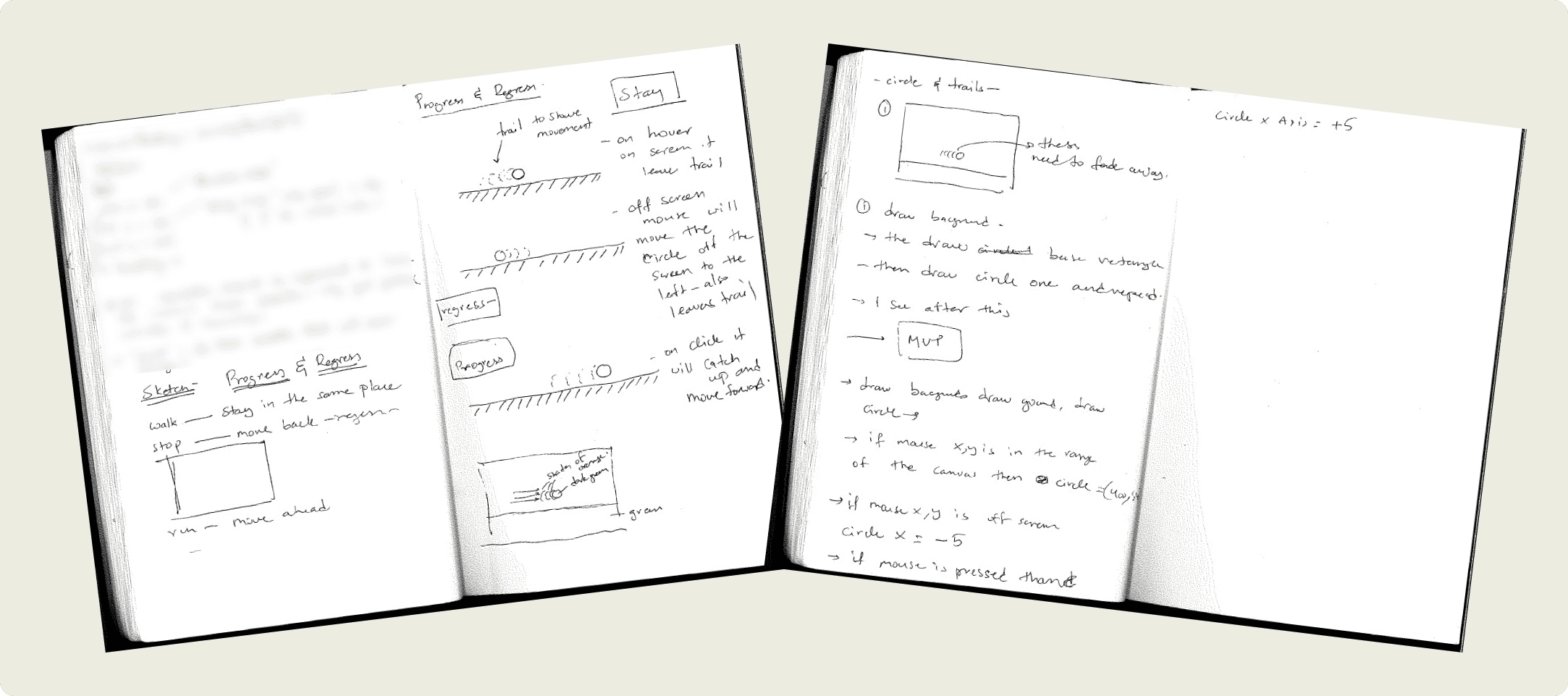

I started with low-fidelity paper sketches to flesh out my idea. These sketches helped me visualize:
The circle's movement
A "floor" element to ground the visual
Basic layout and interaction zones
Higher Fidelity Design
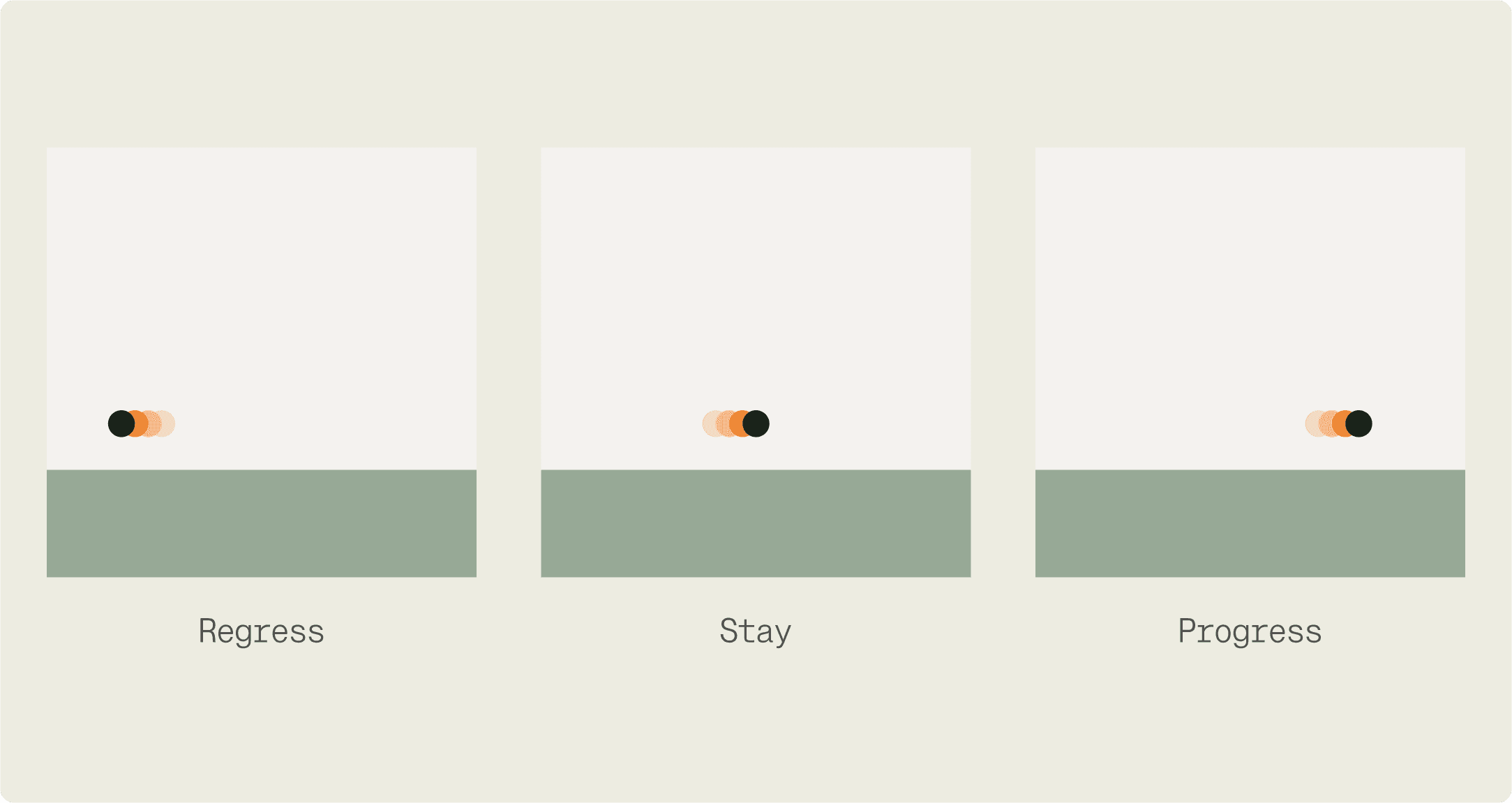

Using Figma, I created a more refined version of my concept, focusing on: Circle positioning, Movement, Color palette and Overall visual feel. This step helped me simplify the design for my MVP, ensuring I could focus on core functionality.
MVP Approach
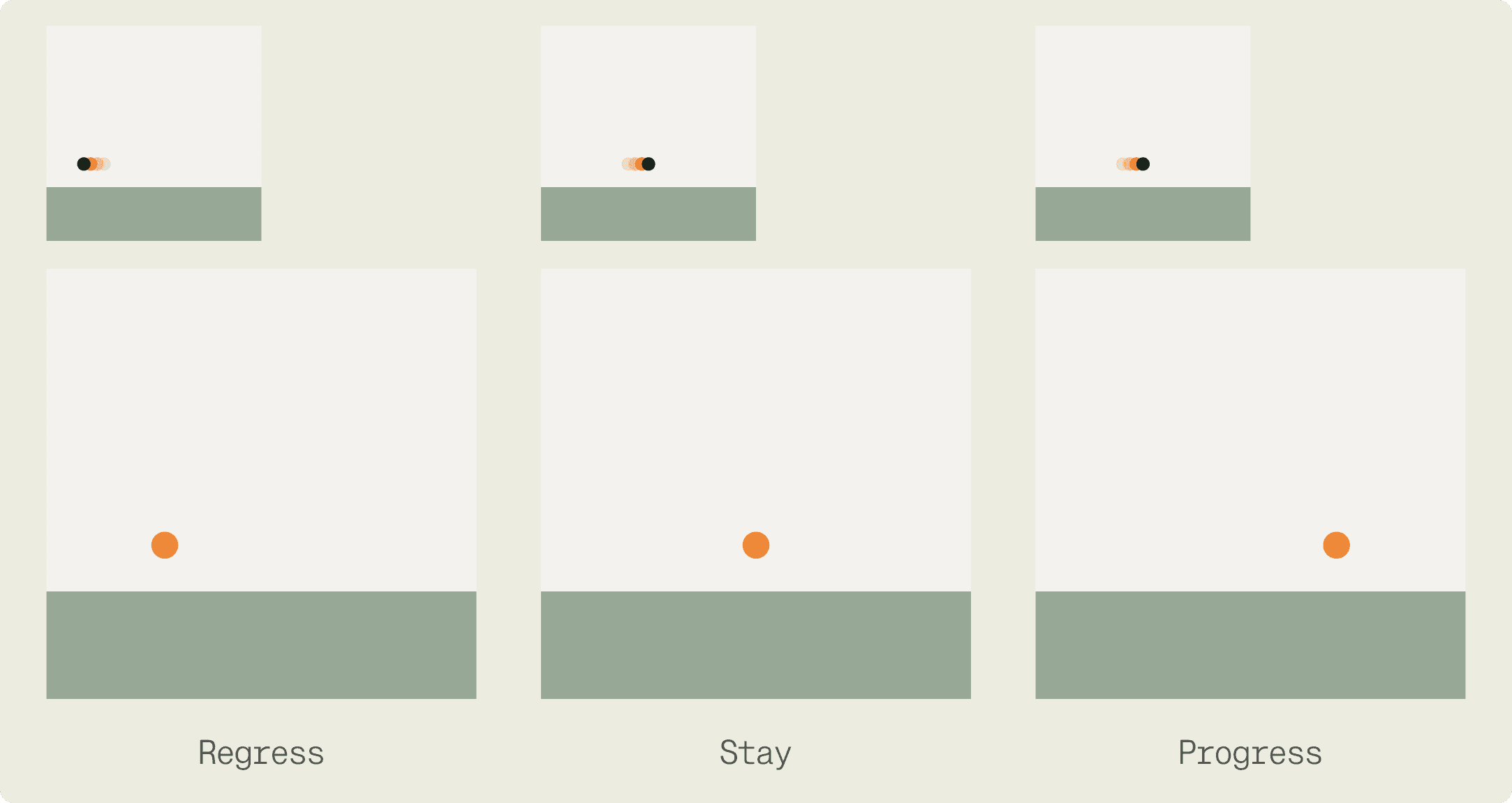
To create a functional prototype quickly, I decided to focus on three key components:
Circle movement based on mouse interaction
Handling different movement states
Conditions for mouse interaction with the canvas
I opted to leave out more complex features like fading trails for this initial version. Although I do have some idea of how we can achieve this by playing around with the alpha value of the circles and having it decrease in relation to speed, I will likely explore this in the next version of the idea or perhaps come back to this blog and update it.
Pseudocode Development
To clarify my logic, I developed pseudocode, which I refined through discussion with my classmate, Nick.
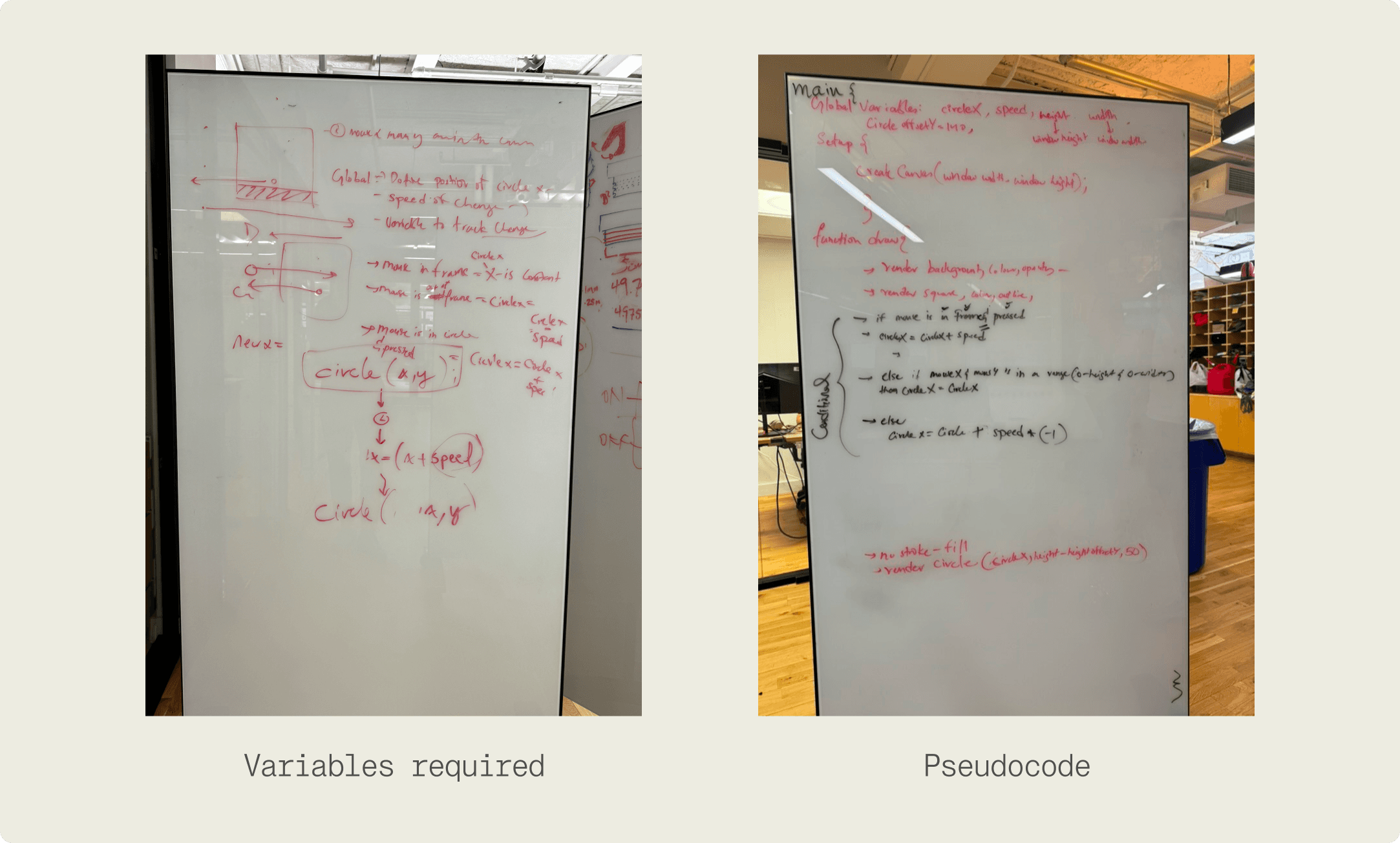

We noted that there was a way to simplify the code further and focus on the variable speed as the primary parameter thats changing so the code was edited to reflect that.
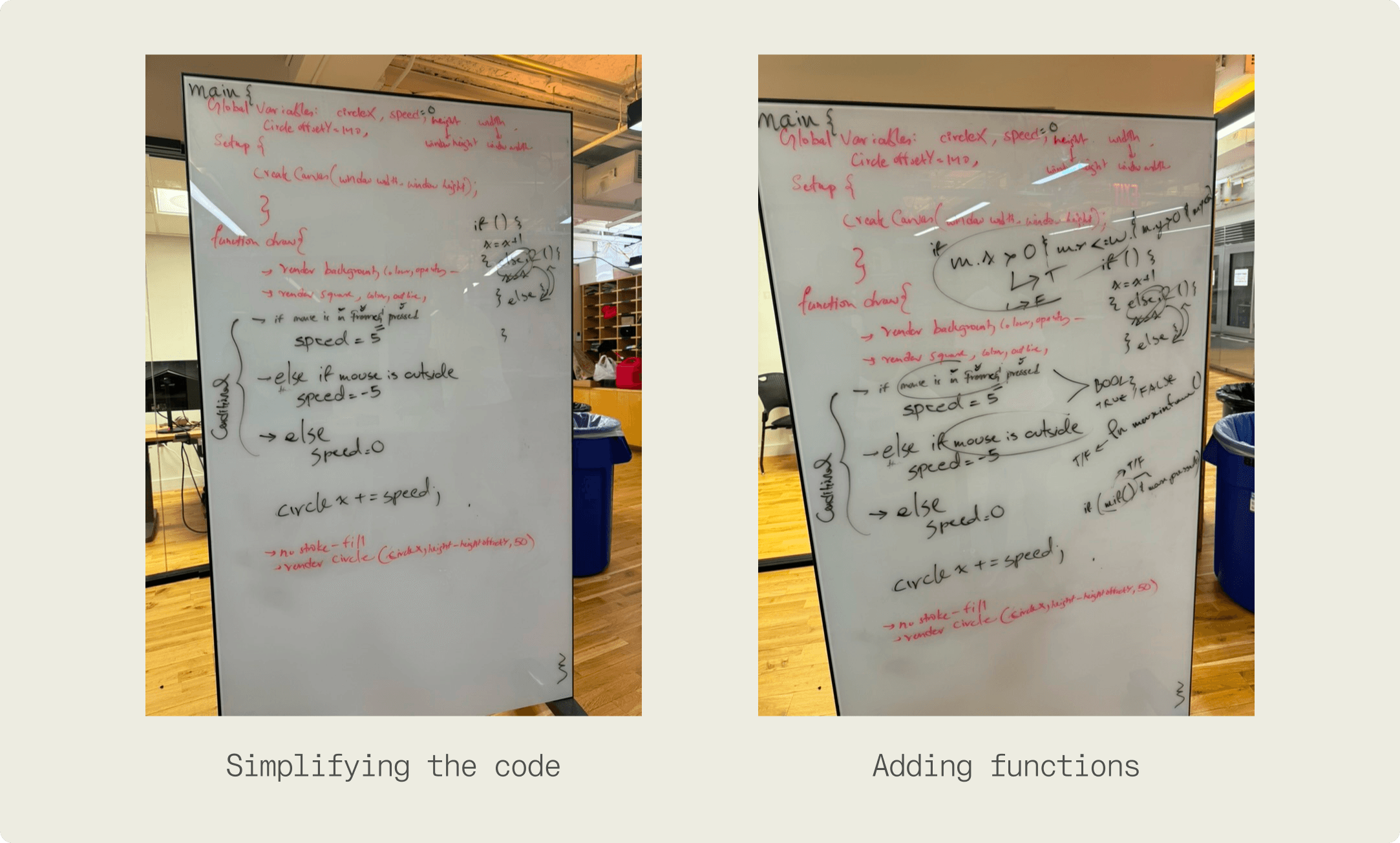
Describing the conditions for position of mouse over and over in the code also was quite tedious so a function was created called inFrame, that would be called on when going through the conditionals. since calling on the function inFrame() would only check if its true, I learned that by adding an exclamation mark you can also check to see if the function is false.
Code Implementation
Translating our pseudocode into P5.js, here's the core of our sketch:
Final Sketch
