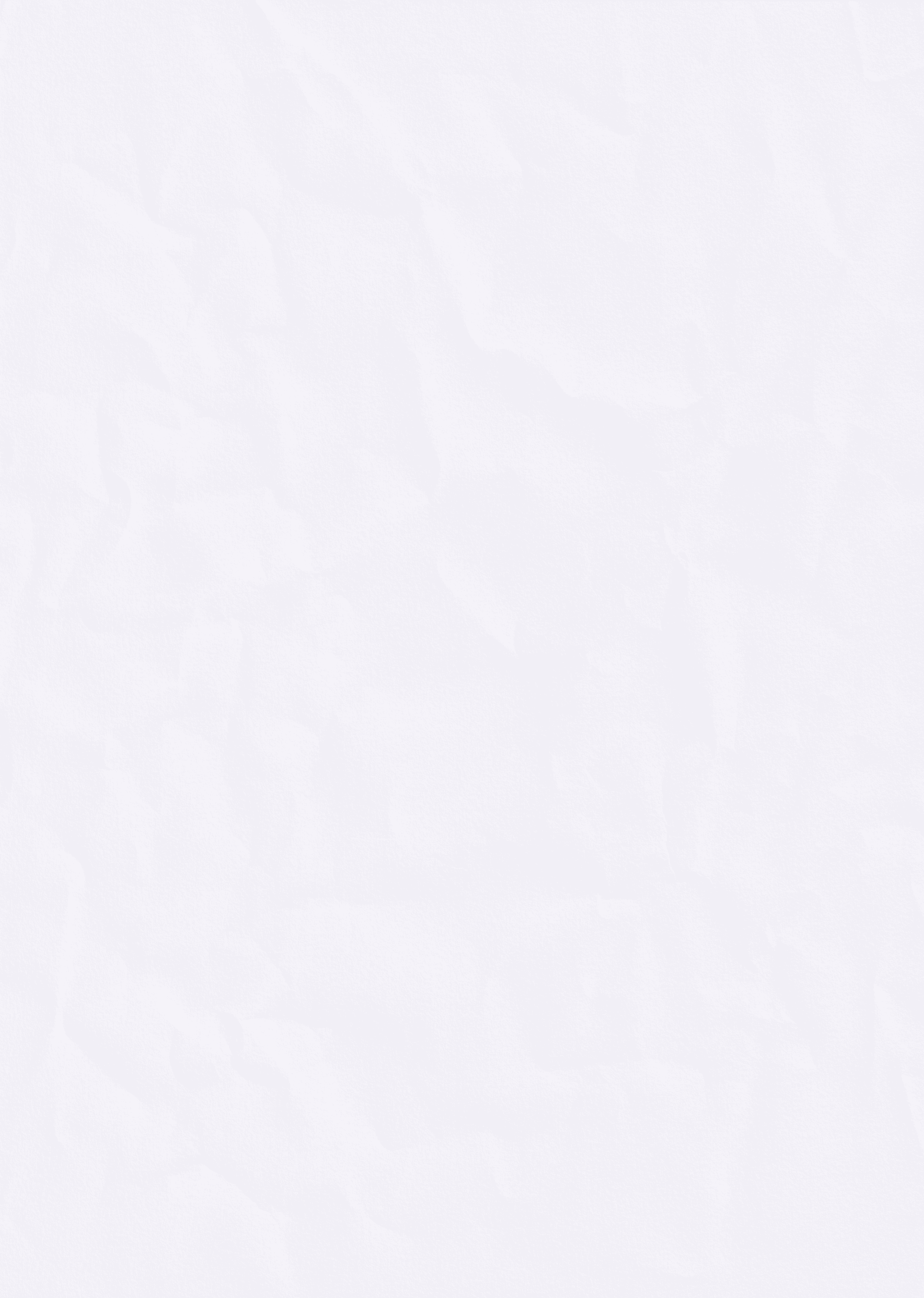
I fixed my Nano Controller (Sort of) // PCOMP W2
Sep 16, 2024
Fixing My Short-Circuited Microcontroller
Last week, I short-circuited my microcontroller and had to go through the process of troubleshooting and repair with Robert. Here’s what we did:
1. Identifying the Problem
We began by checking for continuity across different points on the microcontroller using a multimeter. Initially, we thought two components near the ground pin and USB input were resistors, but we later realized they were capacitors that had failed.
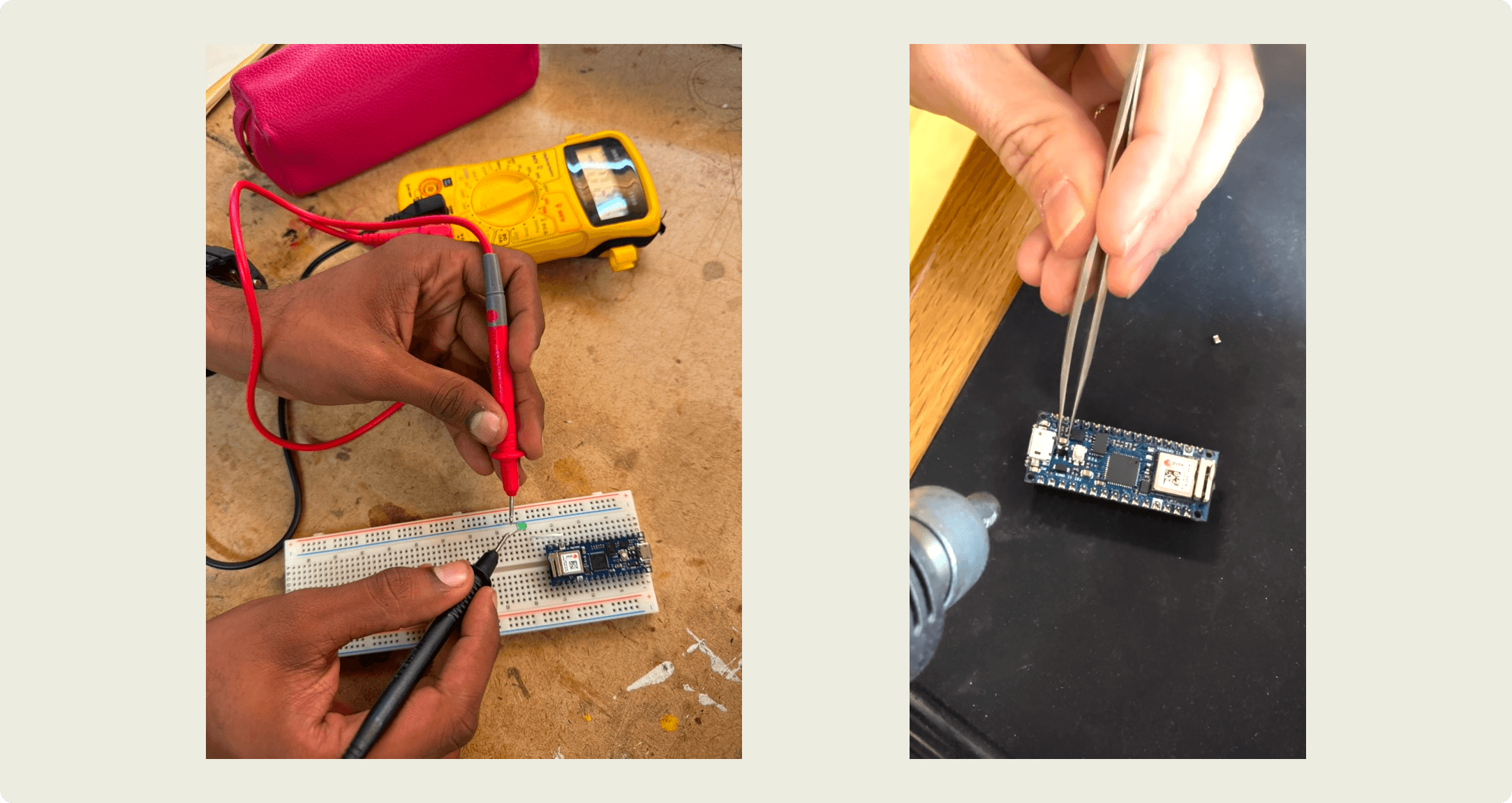
2. Analyzing the Schematic
Using the schematic (shown below), we located the problematic components near the ground pin and USB input. This helped us narrow down the exact capacitors that needed replacing.
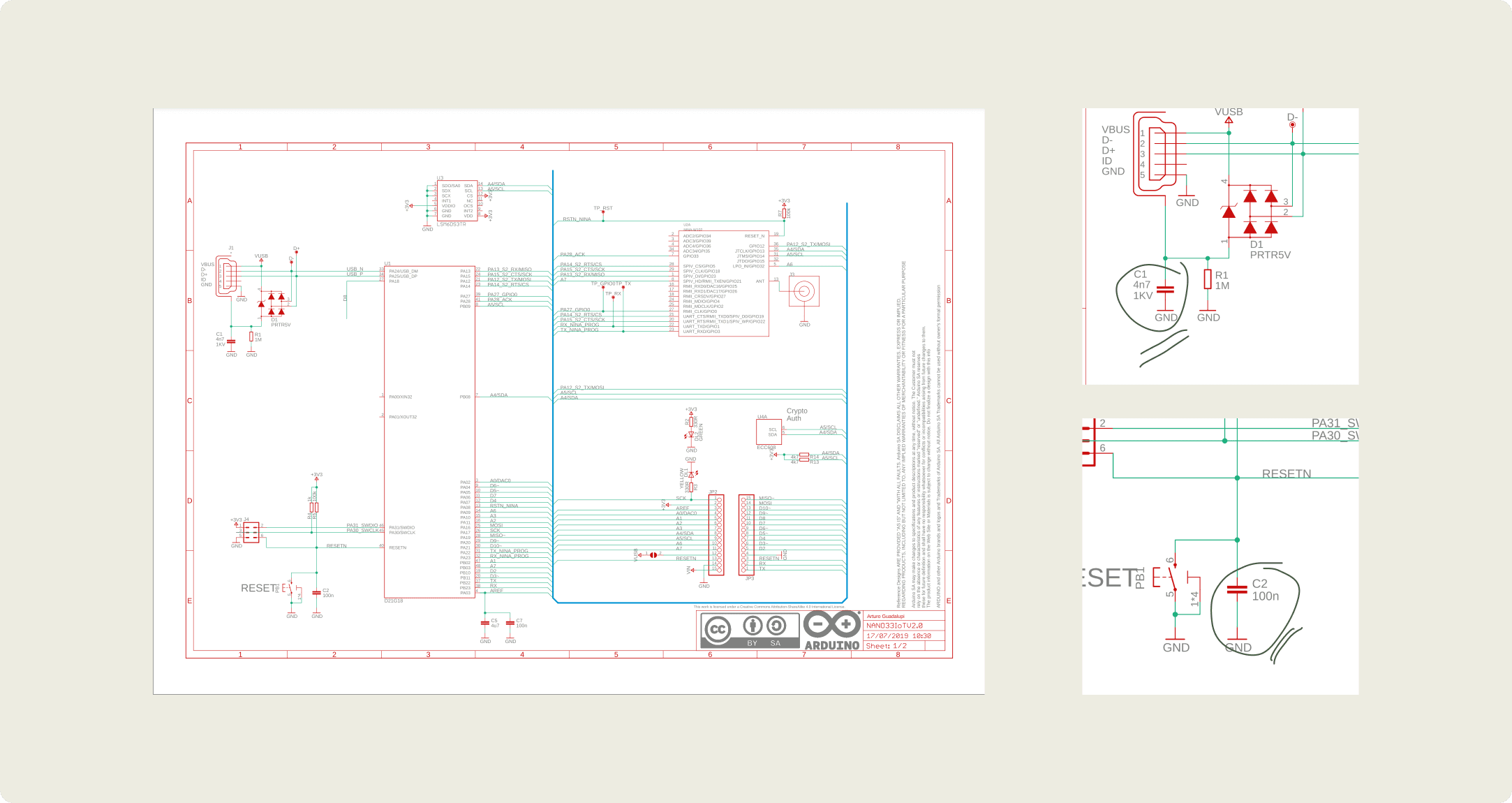
3. Replacing the Capacitors
After identifying the faulty capacitors, we matched their values with what was available in the lab. We replaced them, but at first, the microcontroller still overheated. This led us to reevaluate our approach and find better capacitor matches.
4. Partial Success and Lessons Learned
Once the capacitors were replaced, the microcontroller powered up again, but the lights began flickering. We believe this was due to the capacitors not holding the exact amount of charge required by the Arduino Nano. While not perfect, this was a valuable learning experience in reading schematics and troubleshooting hardware.

Lab 1 : Programming the Arduino
This Lab was straightforward, learning C syntax was new but with the Arduino website reference I was able to manage.
Below is the code for Lab 1 :

Lab 2 : Analog Input
Easy peeezy no trouble at all. I'm also talking through the process in the video so do watch.

Lab 2.1 : FSR
This lab was also pretty straightforward what I also enjoyed was figuring out how the ports relate to each other on from Arduino nano and Arduino uno.

Lab 3.0 : PushButton Change State
This lab was also pretty straightforward what I also enjoyed was figuring out how the ports relate to each other on from Arduino nano and Arduino uno.
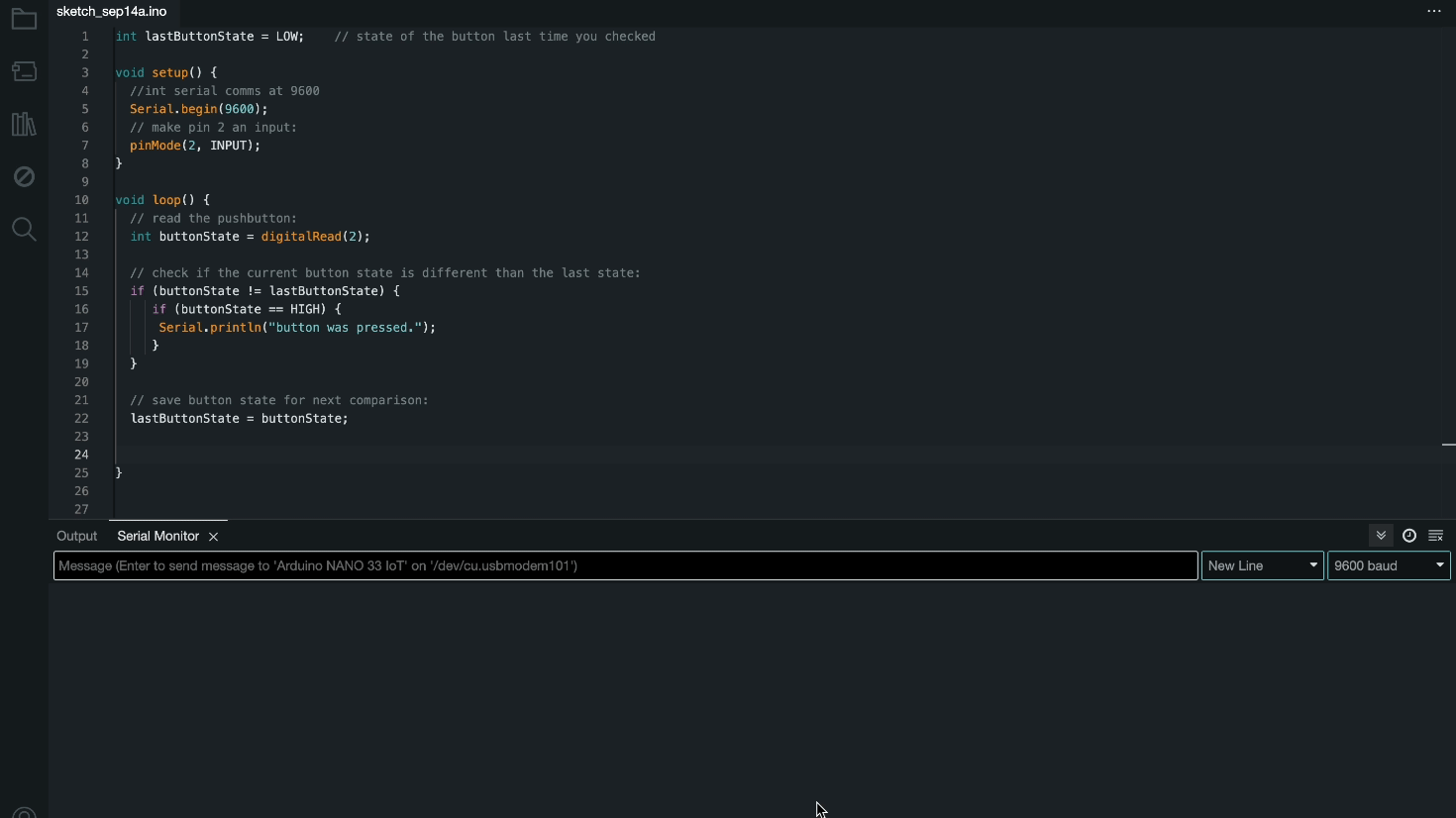

Lab 3.1: Count Button Presses
Most of this was fairly straightforward too.
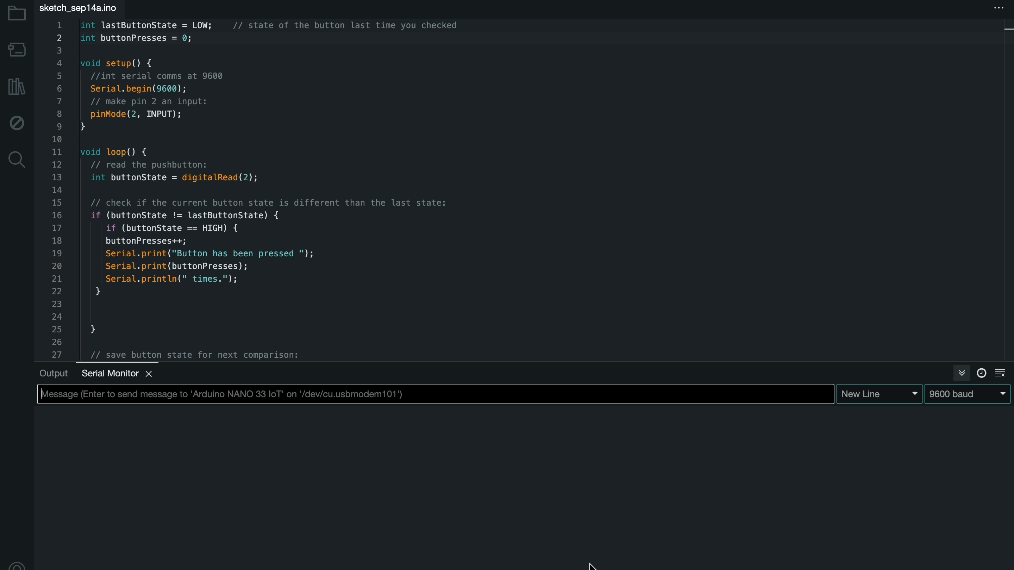
Lab 3.3: Long Press, Short Press
Most of this was fairly straightforward too. I also named my arduino files terribly so I do not have the code for this but heres the gif below.
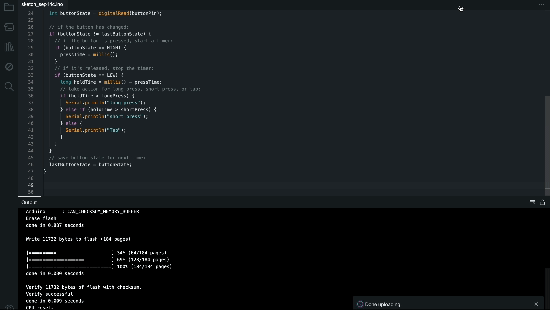
Lab 3.4: Sensor Threshold Crossing
Most of this was fairly straightforward too. I also named my arduino files terribly so I do not have the code for this but heres the gif below.
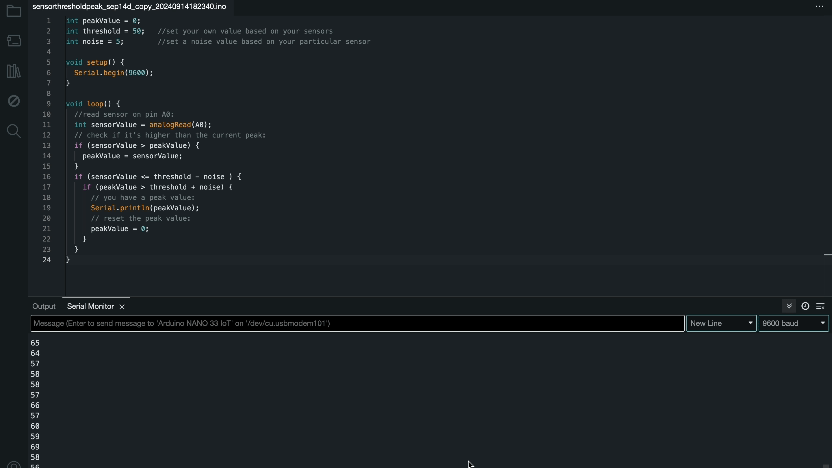
I also did the rest but unfortunately I did not save the code and wrote over the same files as sensor threshold crossing.